Initial Setup
First, go to iTunes Connect and select your app, and then click features. It should look something like this: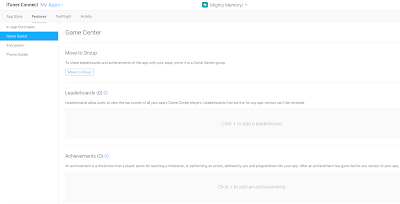
Then click on the plus next to leaderboards and select "Single Leaderboard". You should now be at a page that looks like this:
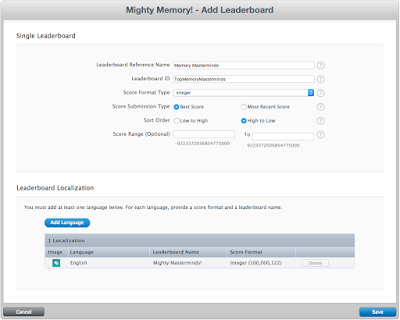
Fill out the fields, the Leaderboard Id will be how you reference it in the code. Ensure you also add a language, the name here will be the name that appears to users viewing your scoreboard:
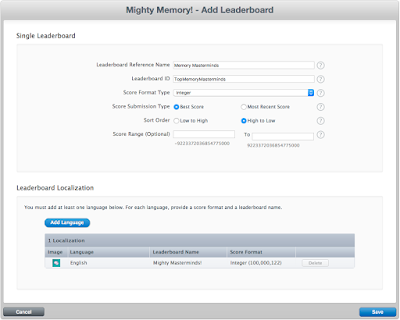
Once you are done click save, and ensure that Game Center is enabled for your app against the build you are setting up. Then you will need to also enable it via Xcode. Open Xcode and ensure that GameKit is added as a Linked Framework/Library:
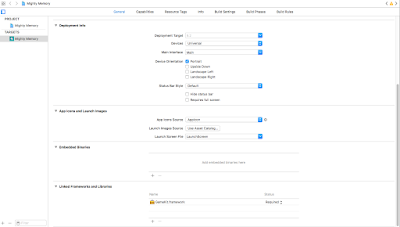
Finally, check the capabilities to ensure Game Center is successfully enabled:

Coding a Leaderboard
First, ensure the user is logged in when they first open the application by authenticating the player in the viewDidLoad function on one of the initial ViewController's presented by the application. Ensure the state of whether game center is enabled or not is saved, as this will allow you whether to show the option to view leaderboard after a user has played your game. For convenience and code simplicity, gameCenterEnabled is a global variable in this example.import UIKit import GameKit class ViewController: UIViewController { override func viewDidLoad() { super.viewDidLoad() score = 1 // Do any additional setup after loading the view, // typically from a nib. authenticateLocalPlayer() } override func didReceiveMemoryWarning() { super.didReceiveMemoryWarning() } func authenticateLocalPlayer() { let localPlayer = GKLocalPlayer.localPlayer() localPlayer.authenticateHandler = {(ViewController, error) -> Void in if((ViewController) != nil) { // 1 Show login if player is not logged in self.present(ViewController!, animated: true, completion: nil) } else if (localPlayer.isAuthenticated) { // 2 Player is already authenticated & logged in gameCenterEnabled = true } else { // 3 Game center is not enabled on the users device gameCenterEnabled = false } } } }
Next, you will want a view with a button to show the leaderboard , the view controller for this view should implement GKGameCenterControllerDelegate and handle dismissal and opening of the leaderboard. In this example saving of the score happens here as this view is displayed upon game completion. However, saving of the score can happen anywhere in your application.
import UIKit import GameKit class ResultViewController: UIViewController, GKGameCenterControllerDelegate { @available(iOS 6.0, *) public func gameCenterViewControllerDidFinish(_ gameCenterViewController: GKGameCenterViewController) { gameCenterViewController.dismiss(animated: false, completion: nil) } override func viewDidLoad() { super.viewDidLoad() saveScore() } override func didReceiveMemoryWarning() { super.didReceiveMemoryWarning() } // save score to leaderboard with matching id func saveScore() { if (gameCenterEnabled) { let leaderboardID = "com.tripwire.memorymasterminds" let scoreToRecord = GKScore(leaderboardIdentifier: leaderboardID) scoreToRecord.value = Int64(score) GKScore.report([scoreToRecord], withCompletionHandler: nil) } } // display leaderboard with matching id @IBAction func showLeaderboardClicked(sender: AnyObject) { let gcVC = GKGameCenterViewController() gcVC.gameCenterDelegate = self gcVC.viewState = GKGameCenterViewControllerState .leaderboards gcVC.leaderboardIdentifier = "com.tripwire.memorymasterminds" self.present(gcVC, animated: false, completion: nil) } }
No comments:
Post a Comment